When I was creating a VSTS Git post-commit service hook it became clear fairly quickly that I needed to be able to simulate calls from VSTS using SoapUI. This is what I did.
SoapUI: https://www.soapui.org/downloads/soapui.html
Python script to capture VSTS requests
First thing needed is a basic Python script to log the incoming request so that we can copy these requests to SoapUI to mimic various commit scenarios. Obviously before you can do this you need to setup Python to run on Apache, see my notes: Enable python scripts in Apache
I created the below Python script that creates a logging file that will include a dump of the http request.
Script: /srv/www/webhooks/log_request.py
Log: /var/log/webhooks/webhooks.log
Requests log: /var/log/webhooks/webhooks_requests.log
#!/usr/bin/env python import cgi; import cgitb;cgitb.enable() import logging import sys, json # Logging config logging.basicConfig(level=logging.DEBUG, format='%(asctime)s %(levelname)s %(message)s', filename='/var/log/webhooks/webhooks.log', filemode='a') # Print headers print "Content-Type: text/html" print "" # Print page print("<html>") print("<title>Webhook Capture JSON Request</title>") print("<body>Capture JSON request by Zoyinc.</body>") print("</html>") received_json_data = sys.stdin.read() requestLogFile = open('/var/log/webhooks/webhooks_requests.log',"a+") parsed = json.loads(received_json_data) requestLogFile.write( '============ Start JSON request ===============\n') requestLogFile.write( json.dumps(parsed, sort_keys=True, indent=4) + '\n') requestLogFile.write( '============ End JSON request =================\n\n\n') requestLogFile.close()
Adding a web hook VSTS service hook
Next thing is to send a test request from VSTS to this script. Login to VSTS, select your project, click on “Project settings” then “Service hooks” and add a new “Subscription”:
From the “Service” dialog select “Web Hooks” and click “Next”:
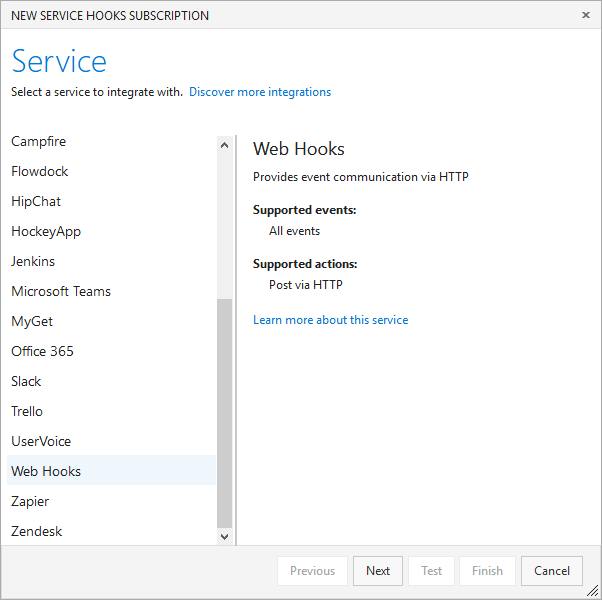
This takes you to the “Trigger” screen from which you can see that should you want this web hook can be restricted to only one specific Git repo and even down to only a particular branch or team. Set your options and click on “Next”:
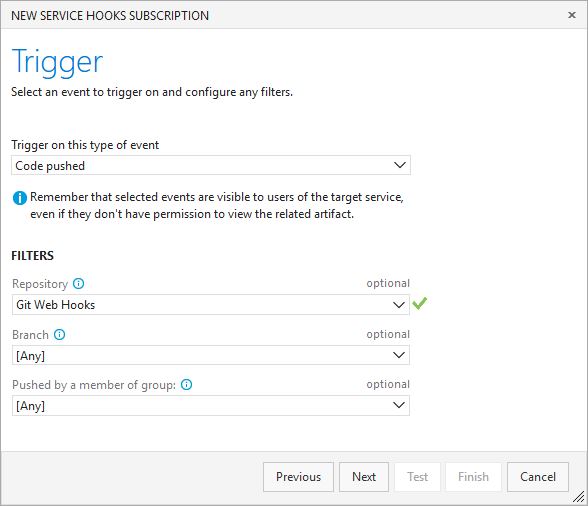
The next screen allows you to define the action to take. For our purposes we accept the defaults and put in the URL of:
http://www.zoyinc.com/webhooks/log_request.py
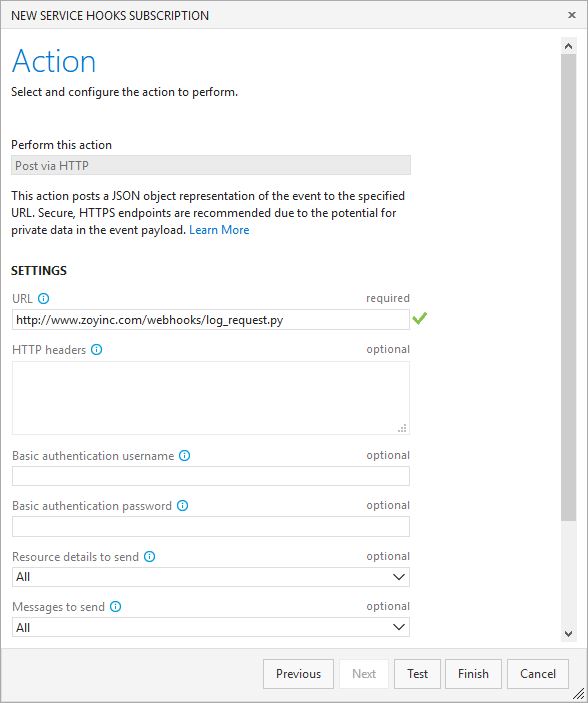
The important thing about this section is that you can send a test message. At the bottom of the “Action” dialog click on the “Test” button and it will send a test commit message to your Python script:
Our test Python script, detailed earlier, will output the requests to a file “/var/log/webhooks/webhooks.log”. Have a look at this file now and you will see the test we sent from VSTS. It will now look something like:
============ Start JSON request =============== { "createdDate": "2018-08-12T06:44:45.3420112Z", "detailedMessage": { "html": "Jamal Hartnett pushed a commit to <a href=\"https://fabrikam-fiber-inc.visualstudio.com/DefaultCollection/_git/Fabrikam-Fiber-Git/\">Fabrikam-Fiber-Git</a>:<a href=\"https://fabrikam-fiber-inc.visualstudio.com/DefaultCollection/_git/Fabrikam-Fiber-Git/#version=GBmaster\">master</a>.\n<ul>\n<li>Fixed bug in web.config file <a href=\"https://fabrikam-fiber-inc.visualstudio.com/DefaultCollection/_git/Fabrikam-Fiber-Git/commit/33b55f7cb7e7e245323987634f960cf4a6e6bc74\">33b55f7c</a>\n</ul>", "markdown": "Jamal Hartnett pushed a commit to [Fabrikam-Fiber-Git](https://fabrikam-fiber-inc.visualstudio.com/DefaultCollection/_git/Fabrikam-Fiber-Git/):[master](https://fabrikam-fiber-inc.visualstudio.com/DefaultCollection/_git/Fabrikam-Fiber-Git/#version=GBmaster).\n* Fixed bug in web.config file [33b55f7c](https://fabrikam-fiber-inc.visualstudio.com/DefaultCollection/_git/Fabrikam-Fiber-Git/commit/33b55f7cb7e7e245323987634f960cf4a6e6bc74)", "text": "Jamal Hartnett pushed a commit to Fabrikam-Fiber-Git:master.\n - Fixed bug in web.config file 33b55f7c" }, "eventType": "git.push", "id": "03c164c2-8912-4d5e-8009-3707d5f83734", "message": { "html": "Jamal Hartnett pushed updates to Fabrikam-Fiber-Git:master.", "markdown": "Jamal Hartnett pushed updates to `Fabrikam-Fiber-Git`:`master`.", "text": "Jamal Hartnett pushed updates to Fabrikam-Fiber-Git:master." }, "notificationId": 9, "publisherId": "tfs", "resource": { "commits": [ { "author": { "date": "2015-02-25T19:01:00Z", "email": "fabrikamfiber4@hotmail.com", "name": "Jamal Hartnett"
}, "collection": { "id": "c12d0eb8-e382-443b-9f9c-c52cba5014c2" }, "project": { "id": "be9b3917-87e6-42a4-a549-2bc06a7a878f" } }, "resourceVersion": "1.0", "subscriptionId": "00000000-0000-0000-0000-000000000000" } ============ End JSON request =================
Thus the section between “============ Start JSON request ===============” and “============ End JSON request =================” is the actual request that we will use with SoapUI to sent test requests.
Now finish the VSTS service hook by closing the test dialog and then clicking on the “Finish” button.
Create SoapUI Project
I am using SoapUI 5.4.0 and it keeps freezing on me. Following a suggestion from Ahmet Aksoy: Soapui GUI freeze in 64 bit Windows , I would suggest prior to starting SoapUI add “-Dsoapui.jxbrowser.disable=true” to the bottom of the file:
C:\Program Files\SmartBear\SoapUI-5.4.0\bin\SoapUI-5.4.0.vmoptions
Moving on …
So now we have our test SoapUI message so open up SoapUI and select:
File | New REST Project
You will be prompted for the URI, enter the URL for your test Python script and click “OK”:
http://www.zoyinc.com/webhooks/log_request.py
You should now see something like belowChange the “Method”, highlighted, from “GET” to “POST”. This will open up a dialog at the bottom where you can paste your request that you captured earlier – ensure you include the start and end curly brackets “{” and “}” as the first and last characters.
Note if you don’t see the “Request 1” panel above then right click on “Request 1” and from the popup menu select “Show Request Editor”:
You should now see something like the below:
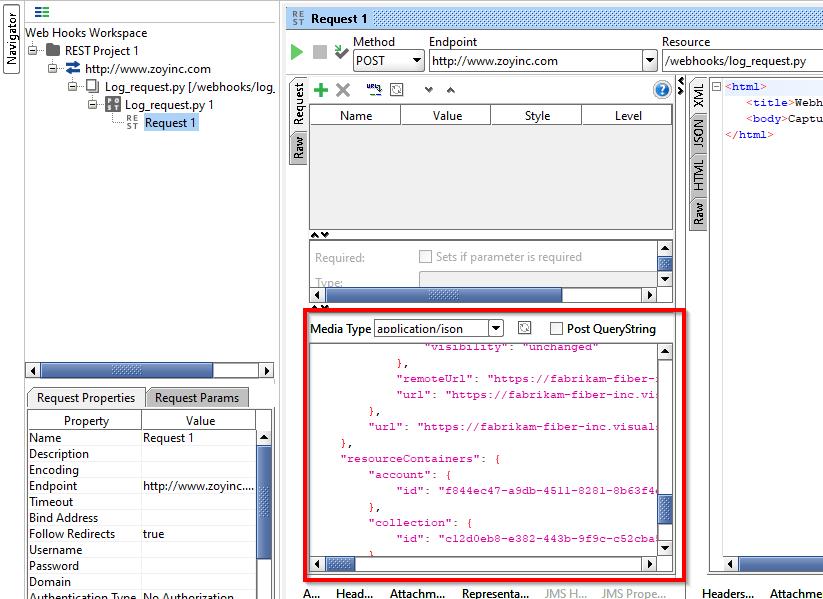
Looking at the highlighted section, you should have “Media Type” set to the default of “application/json” and the text box should be where you pasted the request.
Run the test
To send this test message to your Python test script simply click on the SoapUI “Play” button:
This should send the request which will appear in the file “webhooks_requests.log” as it did earlier when you conducted the test from VSTS. You should compare the contents of the request sent from SoapUI to that sent by VSTS, as recorded in “webhooks_requests.log” – they should be identical.
You should also see the response from the Python script in the results pane:
Done
Now you can mimic VSTS requests and can capture standard commit messages to your repo. Then use these as test requests from SoapUI – meaning you can send the same commit message to your service hook endpoint without having to go through the hassle of actually creating commit after commit after commit.
The sort of tests you might want to run would likely include things like a push which included multiple commits.